Sunday 31 March 2013
Simple Line Drawing In Turbo C Graphics
Well this post consists of the source code for very very simple line drawing using the in-built functions.
I hope it proves useful for learning purpose.
Read more...
#include <stdio.h> #include <conio.h> #include <graphics.h> int main() { int gd = DETECT, gm; initgraph(&gd, &gm, "C:\\TurboC3\\BGI"); line(100, 100, 350, 100); line(100, 100, 70, 140); line(70, 140, 130, 140); line(350, 100, 380, 140); rectangle(70, 140, 130, 200); rectangle(130, 140, 380, 200); getch(); closegraph(); return 0; }
I hope it proves useful for learning purpose.
Read more...
Simple Line Drawing In Turbo C Graphics
2013-03-31T23:00:00+05:45
Cool Samar
c|C/C++|programming|
Comments

Labels:
c,
C/C++,
programming
Bookmark this post:blogger tutorials
Social Bookmarking Blogger Widget | ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
Implementation Of BLA Line Drawing Algorithm
Here is the source code that makes use of the traditional DOS mode graphics to implement the Bresenham line drawing algorithm for the lines with slope |m| < 1.
1) Input two points (x1, y1) & (x2, y2).
2) Determine the differences dx = x2 - x1 and dy = y2 - y1.
3) Calculate the initial decision parameter P0 = 2dy - dx.
4) For each xk along the line starting at k = 0,
if Pk < 0,
a) put a pixel at (xk + 1, yk)
b) Pk+1 = Pk + 2dy
else
a) put a pixel at (xk + 1, yk + 1)
b) Pk+1 = Pk + 2dy - 2dx.
5) Repeat step 4 for dx time.
6) End
Make sure to provide an appropriate path for graphics library.
Read more...
Bresenham Line Drawing Algorithm for |m| < 1
Algorithm
1) Input two points (x1, y1) & (x2, y2).
2) Determine the differences dx = x2 - x1 and dy = y2 - y1.
3) Calculate the initial decision parameter P0 = 2dy - dx.
4) For each xk along the line starting at k = 0,
if Pk < 0,
a) put a pixel at (xk + 1, yk)
b) Pk+1 = Pk + 2dy
else
a) put a pixel at (xk + 1, yk + 1)
b) Pk+1 = Pk + 2dy - 2dx.
5) Repeat step 4 for dx time.
6) End
Source Code
#include <stdio.h> #include <conio.h> #include <graphics.h> #include <math.h> int main() { int gd = DETECT, gm; int x1, y1, x2, y2, dx, dy; int x, y, i, p0, pk; printf("Enter x1, y1: "); scanf("%d %d", &x1, &y1); printf("Enter x2, y2: "); scanf("%d %d", &x2, &y2); dx = x2 - x1; dy = y2 - y1; x = x1; y = y1; p0 = ( 2 * dy - dx); initgraph(&gd, &gm, "C:\\TurboC3\\BGI"); pk = p0; for (i = 0; i < abs(dx); i++) { if (pk < 0) { putpixel(x, y, WHITE); pk += (2 * dy); } else { putpixel(x, y, WHITE); pk += (2 * dy - 2 * dx); } (x1 < x2)?x++:x--; (y1 < y2)?y++:y--; delay(50); } getch(); closegraph(); return 0; }
Make sure to provide an appropriate path for graphics library.
Read more...
Implementation Of BLA Line Drawing Algorithm
2013-03-31T22:05:00+05:45
Cool Samar
c|C/C++|computer graphics|programming|
Comments

Labels:
c,
C/C++,
computer graphics,
programming
Bookmark this post:blogger tutorials
Social Bookmarking Blogger Widget | ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
Tuesday 26 March 2013
Implementation of DDA Line Drawing Algorithm
Here is a source code in traditional Turbo C that makes use of old DOS mode graphics to implement the digital differential analyzer.
2) Determine the differences dx = x2 - x1 and dy = y2 - y1.
3) Choose step size as the bigger value between the absolute values of dx and dy.
4) Determine x-increment = dx/step_size and y-increment = dy/step_size.
5) Start from (x0, y0) = (x1, y1).
6) For i -> 0 to stepsize:
a) draw pixel at (xi, yi)
b) set xk = xk + x-increment
b) set yk = yk + y-increment
Make sure to provide an appropriate path for graphics library.
Read more...
Digital Differential Analyzer
Algorithm
1) Input two points (x1, y1) & (x2, y2).2) Determine the differences dx = x2 - x1 and dy = y2 - y1.
3) Choose step size as the bigger value between the absolute values of dx and dy.
4) Determine x-increment = dx/step_size and y-increment = dy/step_size.
5) Start from (x0, y0) = (x1, y1).
6) For i -> 0 to stepsize:
a) draw pixel at (xi, yi)
b) set xk = xk + x-increment
b) set yk = yk + y-increment
Source Code
#include <stdio.h> #include <conio.h> #include <graphics.h> #include <math.h> int main() { int gd = DETECT, gm; int x1, y1, x2, y2, dx, dy, stepsize; float xinc, yinc, x, y; int i; printf("Enter x1, y1: "); scanf("%d %d", &x1, &y1); printf("Enter x2, y2: "); scanf("%d %d", &x2, &y2); dx = x2 - x1; dy = y2 - y1; stepsize = (abs(dx) > abs(dy))?abs(dx):abs(dy); xinc = dx/(float)stepsize; yinc = dy/(float)stepsize; x = x1; y = y1; initgraph(&gd, &gm, "C:\\TC\\BGI"); putpixel(x, y, WHITE); delay(10); for (i = 0; i < stepsize; i++) { x += xinc; y += yinc; putpixel(x, y, WHITE); delay(50); } getch(); closegraph(); return 0; }
Make sure to provide an appropriate path for graphics library.
Read more...
Implementation of DDA Line Drawing Algorithm
2013-03-26T01:08:00+05:45
Cool Samar
c|C/C++|computer graphics|programming|
Comments

Labels:
c,
C/C++,
computer graphics,
programming
Bookmark this post:blogger tutorials
Social Bookmarking Blogger Widget | ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
Tuesday 19 March 2013
How To View Your Gmail Access History Details
I do this thing on a regular basis to know if my account got compromised or not. Well I understand the risks imposed by logging in to my gmail account but still due to several circumstances, I have to login from public PCs. Though I employ some other techniques to trick possible keyloggers/RATs, etc. I do keep running from the dangers of account hijack and hence keep on regularly checking the account history details in gmail.
In order to access the gmail history log details, you need to scroll down to the right bottom of your gmail inbox where you will notice the option to view the detail of your account which looks like below:
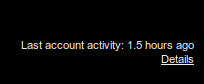
Moreover, it seems like that the details now include the user agents and/or access type information along with the IP address and time of access to the gmail account.
If you're concerned about unauthorized access to your mail, you'll be able to use the data in the 'Access type' column to find out if and when someone accessed your mail. For instance, if the column shows any POP access, but you don't use POP to collect your mail, it may be a sign that your account has been compromised.
For more information, refer to this page.
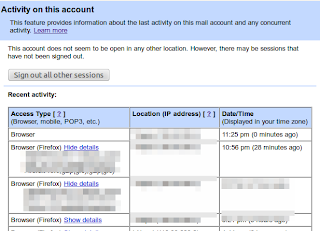
Moreover, this feature lets you log out all of your sessions other than the current session. This can come quite handy whenever you have forgotten to sign out or someone else is having an unauthorized access to your account.
Read more...
In order to access the gmail history log details, you need to scroll down to the right bottom of your gmail inbox where you will notice the option to view the detail of your account which looks like below:
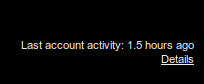
Moreover, it seems like that the details now include the user agents and/or access type information along with the IP address and time of access to the gmail account.
If you're concerned about unauthorized access to your mail, you'll be able to use the data in the 'Access type' column to find out if and when someone accessed your mail. For instance, if the column shows any POP access, but you don't use POP to collect your mail, it may be a sign that your account has been compromised.
For more information, refer to this page.
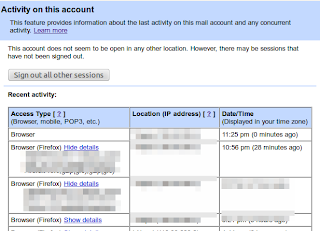
Moreover, this feature lets you log out all of your sessions other than the current session. This can come quite handy whenever you have forgotten to sign out or someone else is having an unauthorized access to your account.
Read more...
How To View Your Gmail Access History Details
2013-03-19T23:38:00+05:45
Cool Samar
gmail|security|
Comments

Bookmark this post:blogger tutorials
Social Bookmarking Blogger Widget | ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
Friday 15 March 2013
Uploaded.net 48 Hours Premium Membership Coupon To Redeem
Hi all, I just bought a Ul.to account and got an extra coupon that lets free users to have 48 hours premium membership. I decided to provided the coupon to one of my readers.
I will provide the coupon to one of the people who share URL of my website in the twitter with mention to me (@techgaun). I'll decide the winner on 16th March. So start tweeting :P (You understand I need something in return for this coupon :) )
Coupon code: UBTIZYMM
Read more...
Coupon code: UBTIZYMM
Read more...
Uploaded.net 48 Hours Premium Membership Coupon To Redeem
2013-03-15T21:59:00+05:45
Cool Samar
giveaway|
Comments

Labels:
giveaway
Bookmark this post:blogger tutorials
Social Bookmarking Blogger Widget | ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
Saturday 9 March 2013
Check Battery Status From Terminal [How To]
Since I had no graphical interface for some reason, I needed some alternative way to check the battery status. If your system includes acpi command, you can just use this command but I had no luxury of such command and here's how you can do the same thing I did.
The /proc/ virtual file system has information of different states among which the ACPI information is one. The ACPI information provides us the details of device configurations and power status of the system. Below is one flavor of the command to check the battery status:
The first command provides the general status of the battery and the second command provides the detailed information about battery. The other way is to use the upower command that talks with the upowerd daemon. Upowerd daemon is a default daemon in ubuntu and few others for power statistics. Below is the command to see battery details:
If you wish to install acpi for future uses, you can do so by typing the command below:
Play around with different switches by looking over the help and man pages. You will find this tool quite useful :)
Read more...
samar@Techgaun:~$ cat /proc/acpi/battery/BAT0/state
present: yes
capacity state: ok
charging state: charged
present rate: unknown
remaining capacity: unknown
present voltage: 12276 mV
samar@Techgaun:~$ cat /proc/acpi/battery/BAT0/info
present: yes
design capacity: 4400 mAh
last full capacity: unknown
battery technology: rechargeable
design voltage: 10800 mV
design capacity warning: 250 mAh
design capacity low: 150 mAh
cycle count: 0
capacity granularity 1: 10 mAh
capacity granularity 2: 25 mAh
model number: Primary
serial number:
battery type: LION
OEM info: Hewlett-Packard
present: yes
capacity state: ok
charging state: charged
present rate: unknown
remaining capacity: unknown
present voltage: 12276 mV
samar@Techgaun:~$ cat /proc/acpi/battery/BAT0/info
present: yes
design capacity: 4400 mAh
last full capacity: unknown
battery technology: rechargeable
design voltage: 10800 mV
design capacity warning: 250 mAh
design capacity low: 150 mAh
cycle count: 0
capacity granularity 1: 10 mAh
capacity granularity 2: 25 mAh
model number: Primary
serial number:
battery type: LION
OEM info: Hewlett-Packard
The first command provides the general status of the battery and the second command provides the detailed information about battery. The other way is to use the upower command that talks with the upowerd daemon. Upowerd daemon is a default daemon in ubuntu and few others for power statistics. Below is the command to see battery details:
samar@Techgaun:~$ upower -i /org/freedesktop/UPower/devices/battery_BAT0
native-path: /sys/devices/LNXSYSTM:00/LNXSYBUS:00/PNP0C0A:00/power_supply/BAT0
vendor: Hewlett-Packard
model: Primary
power supply: yes
updated: Sat Mar 9 10:12:17 2013 (5 seconds ago)
has history: yes
has statistics: yes
battery
present: yes
rechargeable: yes
state: empty
energy: 0 Wh
energy-empty: 0 Wh
energy-full: 47.52 Wh
energy-full-design: 47.52 Wh
energy-rate: 0 W
voltage: 12.28 V
percentage: 0%
capacity: 100%
technology: lithium-ion
native-path: /sys/devices/LNXSYSTM:00/LNXSYBUS:00/PNP0C0A:00/power_supply/BAT0
vendor: Hewlett-Packard
model: Primary
power supply: yes
updated: Sat Mar 9 10:12:17 2013 (5 seconds ago)
has history: yes
has statistics: yes
battery
present: yes
rechargeable: yes
state: empty
energy: 0 Wh
energy-empty: 0 Wh
energy-full: 47.52 Wh
energy-full-design: 47.52 Wh
energy-rate: 0 W
voltage: 12.28 V
percentage: 0%
capacity: 100%
technology: lithium-ion
If you wish to install acpi for future uses, you can do so by typing the command below:
samar@Techgaun:~$ sudo apt-get install acpi
Play around with different switches by looking over the help and man pages. You will find this tool quite useful :)
Read more...
Check Battery Status From Terminal [How To]
2013-03-09T10:15:00+05:45
Cool Samar
linux|tricks and tips|ubuntu|ubuntu 12.04|ubuntu 12.10|
Comments

Labels:
linux,
tricks and tips,
ubuntu,
ubuntu 12.04,
ubuntu 12.10
Bookmark this post:blogger tutorials
Social Bookmarking Blogger Widget | ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
Friday 8 March 2013
Encrypt/Decrypt Confidential Data Using OpenSSL
If you wish to perform encrypted file transfers, openssl provides a robust implementation of SSL v2/3 and TLS v1 as well as full strength generic cryptographic functionalities. Available for almost every commonly used operating system, openssl can be used as a general purpose tool instead of looking for other specialized tools.
If you wish to have full strength cryptographic functions, openssl is a perfect choice. Forget about all other tools that promise to provide high end encryption for your confidential data. Openssl is more than enough for most of your cryptographic needs. Personally, I can't just rely on some random software that promises to provide full strength cryptography but lacks documentations and detailed reviews. Openssl, however, has a well structured documentation and is an open source implementation.
Openssl supports several ciphers such as AES, Blowfish, RC5, etc., several cryptographic hash functions such as MD5, SHA512, etc., and public key cryptographies such as RSA, DSA, etc. Openssl has been widely used in several softwares most notably the OpenSSH.
Now that we know some basics about what OpenSSL is, lets move on encrypting/decrypting files/data using openssl. OpenSSL can take any file and then apply one of the cryptographic functions to encrypt the file. As an example, we encrypt a confidential file 'priv8' with a password "hello" below:
In order to decrypt the encrypted file, we can run the following command:
Now that you know the basic syntax, you can choose among several available cryptographic functions. There are several other symmetric ciphers available for use. The full list of these ciphers is provided by the command:
I hope this helps for your file encryption needs :)
Read more...
If you wish to have full strength cryptographic functions, openssl is a perfect choice. Forget about all other tools that promise to provide high end encryption for your confidential data. Openssl is more than enough for most of your cryptographic needs. Personally, I can't just rely on some random software that promises to provide full strength cryptography but lacks documentations and detailed reviews. Openssl, however, has a well structured documentation and is an open source implementation.
Openssl supports several ciphers such as AES, Blowfish, RC5, etc., several cryptographic hash functions such as MD5, SHA512, etc., and public key cryptographies such as RSA, DSA, etc. Openssl has been widely used in several softwares most notably the OpenSSH.
Now that we know some basics about what OpenSSL is, lets move on encrypting/decrypting files/data using openssl. OpenSSL can take any file and then apply one of the cryptographic functions to encrypt the file. As an example, we encrypt a confidential file 'priv8' with a password "hello" below:
samar@Techgaun:~$ openssl aes-256-cbc -e -in priv8 -out priv8.enc -pass pass:hello
In order to decrypt the encrypted file, we can run the following command:
samar@Techgaun:~$ openssl aes-256-cbc -e -in priv8.enc -out priv8 -pass pass:hello
Now that you know the basic syntax, you can choose among several available cryptographic functions. There are several other symmetric ciphers available for use. The full list of these ciphers is provided by the command:
samar@Techgaun:~$ openssl list-cipher-algorithms
I hope this helps for your file encryption needs :)
Read more...
Encrypt/Decrypt Confidential Data Using OpenSSL
2013-03-08T22:10:00+05:45
Cool Samar
encryption|filesystem|security|
Comments

Labels:
encryption,
filesystem,
security
Bookmark this post:blogger tutorials
Social Bookmarking Blogger Widget | ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
Thursday 7 March 2013
Make An Encrypted Call On Android Using RedPhone
If you are quite worried about your privacy while making voice calls on your phone, RedPhone is a perfect tool to install in your android phone. RedPhone ensures that the eavesdropping attackers can not sniff your call by providing end-to-end encryption.
RedPhone is an open source communication encryption android software that well-integrates with the system dialer and lets you use the default system dialer and contacts apps to make calls as you normally would. The tool is written by Maxie Morlinspike, the same guy who wrote a famous tool called SSLStrip for performing HTTPS stripping attacks.
It is an open source tool licensed under GPL v3; the github README says, RedPhone is an application that enables encrypted voice communication between RedPhone users. RedPhone integrates with the system dialer to provide a frictionless call experience, but uses ZRTP to setup an encrypted VoIP channel for the actual call. RedPhone was designed specifically for mobile devices, using audio codecs and buffer algorithms tuned to the characteristics of mobile networks, and using push notifications to maximally preserve your device's battery life while still remaining responsive.
If you wish to understand more on Encryption protocol, you should refer to the WIKI.
Read more...
RedPhone is an open source communication encryption android software that well-integrates with the system dialer and lets you use the default system dialer and contacts apps to make calls as you normally would. The tool is written by Maxie Morlinspike, the same guy who wrote a famous tool called SSLStrip for performing HTTPS stripping attacks.
Install RedPhone
It is an open source tool licensed under GPL v3; the github README says, RedPhone is an application that enables encrypted voice communication between RedPhone users. RedPhone integrates with the system dialer to provide a frictionless call experience, but uses ZRTP to setup an encrypted VoIP channel for the actual call. RedPhone was designed specifically for mobile devices, using audio codecs and buffer algorithms tuned to the characteristics of mobile networks, and using push notifications to maximally preserve your device's battery life while still remaining responsive.
If you wish to understand more on Encryption protocol, you should refer to the WIKI.
Install RedPhone
Read more...
Make An Encrypted Call On Android Using RedPhone
2013-03-07T21:16:00+05:45
Cool Samar
android|mobile|security|web|
Comments

Bookmark this post:blogger tutorials
Social Bookmarking Blogger Widget | ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
E-Paath - A Perfect Computer-based Learning Tool For Your Children
If you are looking for good computer based learning materials for your small children, e-paaath (E-/Online Lesson) is a perfect choice esp. for the Nepali education scenario. I don't know how much has been done to take this software to the reach of the students/children but I think this software can be a very useful interactive learning material for children.
Developed by OLE Nepal in collaboration with the Department of Education (Nepal), this web-based software provides several modules of online lessons for classes 2-6. The software consists of 18-30 lessons organized in a weekly fashion for four subjects: Nepali, English, Mathematics, and Science. The contents for science are available in both English and Nepali languages. However, mathematics is available only in Nepali language.
E-paath is a flash based content and hence requires flash player and can be run through any of the major web browsers such as Mozilla Firefox, Google Chrome, etc. Since e-paath is a web based content, you can run it in any platform without any problem (I had to change a little bit of code in karma.html file to run the tool smoothly in Linux but its still fine; having a web server to serve the pages solves all errors though).
You can download e-paath from HERE. For installation help, you can refer to this page. You can also access the software online from HERE. Btw, there is no specifically linux version of tool available in the website (except for Sugar desktop environment) and don't try to mirror the online version of e-paath as flash contents seem to be internally referencing the configuration files. Your best bet is to download either of the two available versions and then delete all the unnecessary stuffs in there. It just runs fine.
Read more...
Developed by OLE Nepal in collaboration with the Department of Education (Nepal), this web-based software provides several modules of online lessons for classes 2-6. The software consists of 18-30 lessons organized in a weekly fashion for four subjects: Nepali, English, Mathematics, and Science. The contents for science are available in both English and Nepali languages. However, mathematics is available only in Nepali language.

E-paath is a flash based content and hence requires flash player and can be run through any of the major web browsers such as Mozilla Firefox, Google Chrome, etc. Since e-paath is a web based content, you can run it in any platform without any problem (I had to change a little bit of code in karma.html file to run the tool smoothly in Linux but its still fine; having a web server to serve the pages solves all errors though).
You can download e-paath from HERE. For installation help, you can refer to this page. You can also access the software online from HERE. Btw, there is no specifically linux version of tool available in the website (except for Sugar desktop environment) and don't try to mirror the online version of e-paath as flash contents seem to be internally referencing the configuration files. Your best bet is to download either of the two available versions and then delete all the unnecessary stuffs in there. It just runs fine.
Read more...
E-Paath - A Perfect Computer-based Learning Tool For Your Children
2013-03-07T13:54:00+05:45
Cool Samar
educational material|useful website|web|
Comments

Labels:
educational material,
useful website,
web
Bookmark this post:blogger tutorials
Social Bookmarking Blogger Widget | ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
Subscribe to:
Posts (Atom)